5. Eating the dots and receiving points.
If we want to "eat" the dots, we must check "collisions" between our character and them. As we are using text mode, collissions are simple: both elements will be in the same position on screen, so their X and Y coordinates must be the same: "if ( (x==xDot1) && (y==yDot2) ) ...;"
When this happens, we will give 10 points to the player (so we will need a new variable named "score"):
/* First mini-console-game skeleton Version G: Score (first approach) */ using System; public class Game01g { public static void Main() { int x=40, y=12; int xDot1=10, yDot1=5; int xDot2=20, yDot2=15; int xDot3=60, yDot3=11; int score = 0; ConsoleKeyInfo userKey; // Game Loop while( 1 == 1 ) { // Draw Console.Clear(); Console.Write("Score: {0}",score); Console.SetCursorPosition(xDot1, yDot1); Console.Write("."); Console.SetCursorPosition(xDot2, yDot2); Console.Write("."); Console.SetCursorPosition(xDot3, yDot3); Console.Write("."); Console.SetCursorPosition(x, y); Console.Write("C"); // Read keys and calculate new position userKey = Console.ReadKey(false); if(userKey.Key == ConsoleKey.RightArrow) x++; if(userKey.Key == ConsoleKey.LeftArrow) x--; if(userKey.Key == ConsoleKey.DownArrow) y++; if(userKey.Key == ConsoleKey.UpArrow) y--; // Move enemies and environment // TO DO // Collisions, lose energy or lives, etc if (((x == xDot1) && (y == yDot1)) || ((x == xDot2) && (y == yDot2)) || ((x == xDot3) && (y == yDot3)) ) score += 10; // Pause till next fotogram // TO DO } } }
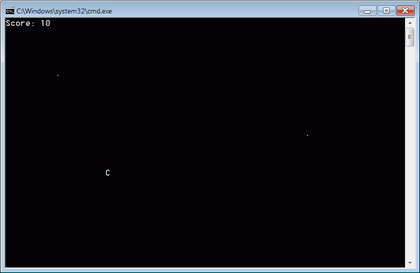
Suggested exercise: Improve this version of the game, so that it ends when the user gets 30 points.