4. Three dots on screen.
The Pac-man game consists on eating dots, or pellets, while avoiding certain enemies (phantoms, ghosts or monsters). Because of this, we are going to start by drawing three dots on screen, and in the next part of this tutorial we will be able to "eat" them and get points from them.
This means several simple changes:
- If we want to have three dots, we will three new coordinates X and three new coordinates Y (we will see alternative ways to do it, less repetitive, later) We might call them sDot1, xDot2 and so on.
- When we draw the game elements on screen, we will not only show our character, but also these dots.
Our program might become:
/* First mini-console-game skeleton Version F: Three dots on screen */ using System; public class Game01f { public static void Main() { int x=40, y=12; int xDot1=10, yDot1=5; int xDot2=20, yDot2=15; int xDot3=60, yDot3=11; ConsoleKeyInfo userKey; // Game Loop while( 1 == 1 ) { // Draw Console.Clear(); Console.SetCursorPosition(xDot1, yDot1); Console.Write("."); Console.SetCursorPosition(xDot2, yDot2); Console.Write("."); Console.SetCursorPosition(xDot3, yDot3); Console.Write("."); Console.SetCursorPosition(x, y); Console.Write("C"); // Read keys and calculate new position userKey = Console.ReadKey(false); if(userKey.Key == ConsoleKey.RightArrow) x++; if(userKey.Key == ConsoleKey.LeftArrow) x--; if(userKey.Key == ConsoleKey.DownArrow) y++; if(userKey.Key == ConsoleKey.UpArrow) y--; // Move enemies and environment // TO DO // Collisions, lose energy or lives, etc // TO DO // Pause till next fotogram // TO DO } } }
And on screen it would be:
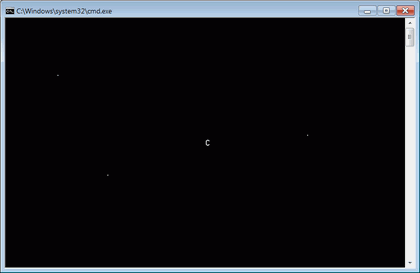
Suggested exercise: Improve this version of the game, so that dots are not placed in fixed positions, but in random ones.