13. Graphic mode: real images
It's time for our game to start showing "real images". We will use a ready-made skeleton, which will allow us not to have to deal with low-level graphic libraries yet:
This way, our program will look quite similar to what it was before. For example, we will show an image in certain coordinates using:
SdlHardware.DrawHiddenImage(pacImage, x, y);
(Why "HiddenImage"? Because we will draw all the images in a hidden screen and finally dump them all at a time to the visible screen; this is called "using a double buffer", and will help us avoid flickering).
And we will check the keyboard with:
if (SdlHardware.KeyPressed(SdlHardware.KEY_RIGHT)) x+=pacSpeed;
Before that, we must enter the graphics mode, indicating the desired width and height (for example 800x600), the color-depth (bits per pixel, typically 24) and if we want our game to run full-screen or windowed:
bool fullScreen = false; SdlHardware.Init(800, 600, 24, fullScreen);
And we must also load each imagen that we will use:
Image pacImage = new Image("pac01.bmp");
The whole source file would be:
/* First mini-graphics-game skeleton Version A (based on console version M: arrays for dots, random positions) */ using System; public class Game02a { public static void Main() { bool fullScreen = false; SdlHardware.Init(800, 600, 24, fullScreen); Image dotImage = new Image("dot.bmp"); Image enemyImage = new Image("ghostGreen.bmp"); Image pacImage = new Image("pac01r.bmp"); int x=40, y=12; int pacSpeed = 6; int amountOfDots = 100; int[] xDot = new int[amountOfDots]; int[] yDot = new int[amountOfDots]; bool[] visible = new bool[amountOfDots]; Random randomNumberGenerator = new Random(); for(int i=0; i<amountOfDots; i++) { xDot[i] = randomNumberGenerator.Next(0,760); yDot[i] = randomNumberGenerator.Next(40,560); visible[i] = true; } int amountOfEnemies = 4; float[] xEnemy = { 150, 400, 500, 600 }; float[] yEnemy = { 100, 200, 300, 400 }; float[] incrXEnemy = { 5f, 3f, 6f, 4.5f }; int score = 0; // Game Loop while( 1 == 1 ) { // Draw SdlHardware.ClearScreen(); //Console.Write("Score: {0}",score); for(int i=0; i<amountOfDots; i++) { if (visible[i]) SdlHardware.DrawHiddenImage(dotImage, xDot[i], yDot[i]); } SdlHardware.DrawHiddenImage(pacImage, x, y); for(int i=0; i<amountOfEnemies; i++) SdlHardware.DrawHiddenImage(enemyImage, (int)xEnemy[i], (int)yEnemy[i]); SdlHardware.ShowHiddenScreen(); // Read keys and calculate new position if (SdlHardware.KeyPressed(SdlHardware.KEY_RIGHT)) x+=pacSpeed; if (SdlHardware.KeyPressed(SdlHardware.KEY_LEFT)) x-=pacSpeed; if (SdlHardware.KeyPressed(SdlHardware.KEY_DOWN)) y+=pacSpeed; if (SdlHardware.KeyPressed(SdlHardware.KEY_UP)) y-=pacSpeed; // Move enemies and environment for(int i=0; i<amountOfEnemies; i++) { xEnemy[i] += incrXEnemy[i]; if ((xEnemy[i] < 1) || (xEnemy[i] > 760)) incrXEnemy[i] = -incrXEnemy[i]; } // Collisions, lose energy or lives, etc for(int i=0; i<amountOfDots; i++) if ((x == xDot[i]) && (y == yDot[i]) && visible[i]) { score += 10; visible[i] = false; } // Pause till next fotogram SdlHardware.Pause(40); } } }
And we would have this appearance on screen:
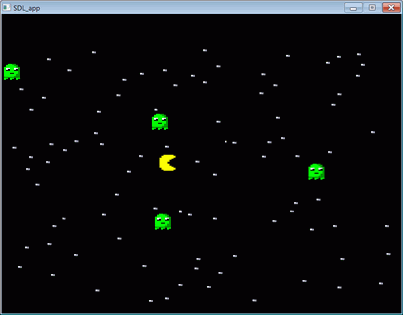
If you want to download the whole project, including images, DLL
files and the auxiliary "SdlHardware.cs" file, you can find them in the "Google Code"
page for this project. This version is called "SdlMuncher001.zip":
code.google.com/p/sdl-muncher/