11. Lots of dots in random positions
Creating lots of dots is not difficult. We can use arrays, as we did for the enemies, but we might prefer not to have fixed positions, but random ones.
In this case, we would create the arrays with no default values:
int amountOfDots = 100; int[] xDot = new int[amountOfDots]; int[] yDot = new int[amountOfDots]; bool[] visible = new bool[amountOfDots];
And we would use a random number generator, to assign values from 0 to 79 for the X coordinate and from 1 to 24 for the Y coordinate:
Random randomNumberGenerator = new Random(); for(int i=0; i<amountOfDots; i++) { xDot[i] = randomNumberGenerator.Next(0,80); yDot[i] = randomNumberGenerator.Next(1,25); visible[i] = true; }
The other changes are barely "for" statements to get the values
from the arrays:
/* First mini-console-game skeleton Version M: arrays for dots, random positions */ using System; using System.Threading; // For the pause with Thread.Sleep public class Game01m { public static void Main() { int x=40, y=12; int amountOfDots = 100; int[] xDot = new int[amountOfDots]; int[] yDot = new int[amountOfDots]; bool[] visible = new bool[amountOfDots]; Random randomNumberGenerator = new Random(); for(int i=0; i<amountOfDots; i++) { xDot[i] = randomNumberGenerator.Next(0,80); yDot[i] = randomNumberGenerator.Next(1,25); visible[i] = true; } int amountOfEnemies = 4; float[] xEnemy = { 15, 40, 50, 60 }; float[] yEnemy = { 5, 10, 15, 20 }; float[] incrXEnemy = { 0.4f, 0.35f, 0.6f, 0.3f }; int score = 0; ConsoleKeyInfo userKey; // Game Loop while( 1 == 1 ) { // Draw Console.Clear(); Console.Write("Score: {0}",score); for(int i=0; i<amountOfDots; i++) { if (visible[i]) { Console.SetCursorPosition(xDot[i], yDot[i]); Console.Write("."); } } Console.SetCursorPosition(x, y); Console.Write("C"); for(int i=0; i<amountOfEnemies; i++) { Console.SetCursorPosition((int)xEnemy[i], (int)yEnemy[i]); Console.Write("A"); } // Read keys and calculate new position if (Console.KeyAvailable) { userKey = Console.ReadKey(false); if(userKey.Key == ConsoleKey.RightArrow) x++; if(userKey.Key == ConsoleKey.LeftArrow) x--; if(userKey.Key == ConsoleKey.DownArrow) y++; if(userKey.Key == ConsoleKey.UpArrow) y--; } // Move enemies and environment for(int i=0; i<amountOfEnemies; i++) { xEnemy[i] += incrXEnemy[i]; if ((xEnemy[i] < 1) || (xEnemy[i] > 78)) incrXEnemy[i] = -incrXEnemy[i]; } // Collisions, lose energy or lives, etc for(int i=0; i<amountOfDots; i++) if ((x == xDot[i]) && (y == yDot[i]) && visible[i]) { score += 10; visible[i] = false; } // Pause till next fotogram Thread.Sleep(40); } } }
And on screen we would get:
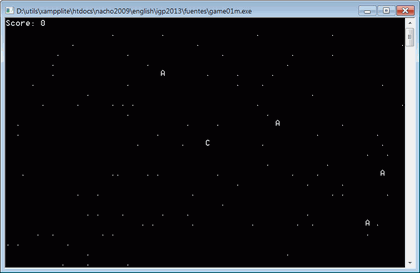