7. A moving enemy
Making an enemy move is not very difficult: we must prepare its "x" and "y" coordinates (we might call them xEnemy and yEnemy), we must draw in the "drawing part" of the "game loop", and we must change its "x" and "y" in the "moving elements" block.
The simplest way to change its position is to change only its "x" coordinate (for example), by increasing it one unit in each frame. We would do it by "x = x + 1;" which can be abbreviated "x++;"
But if we always increase the value of "x", we will soon go out of the visible screen, and this can cause the enemy to seem invisible or even the program to crash (in most games libraries, like the one we will use later, we can write out of the screen freely, but not in the "console" we're using so far.
Because of it, we will check if we reached the end of the screen, to make the enemy then "bounce" and return in the opposite direction
There are several ways to achieve this. In most cases, they mean not to always add 1 to the "x" coordinate, but an "increment", which will start being 1 (so that the enemy moves to the right) and sometimes we will change it to -1 (so that it returns to the left).
We might do it with
if (xe1 == 0) incr1 = 1; if (xe1 == 79) incr1 = -1;
or, in only one condition, with:
if ((xe1 == 0) || (xe1 == 79)) incr1 = - incr1;
In this example, we will do it the second way, so the complete game might look like this:
/* First mini-console-game skeleton Version I: moving enemy */ using System; public class Game01i { public static void Main() { int x=40, y=12; int xDot1=10, yDot1=5; bool visibleDot1 = true; int xDot2=20, yDot2=15; bool visibleDot2 = true; int xDot3=60, yDot3=11; bool visibleDot3 = true; int xEnemy=15, yEnemy=17; int incrXEnemy=1; int score = 0; ConsoleKeyInfo userKey; // Game Loop while( 1 == 1 ) { // Draw Console.Clear(); Console.Write("Score: {0}",score); if (visibleDot1) { Console.SetCursorPosition(xDot1, yDot1); Console.Write("."); } if (visibleDot2) { Console.SetCursorPosition(xDot2, yDot2); Console.Write("."); } if (visibleDot3) { Console.SetCursorPosition(xDot3, yDot3); Console.Write("."); } Console.SetCursorPosition(x, y); Console.Write("C"); Console.SetCursorPosition(xEnemy, yEnemy); Console.Write("A"); // Read keys and calculate new position userKey = Console.ReadKey(false); if(userKey.Key == ConsoleKey.RightArrow) x++; if(userKey.Key == ConsoleKey.LeftArrow) x--; if(userKey.Key == ConsoleKey.DownArrow) y++; if(userKey.Key == ConsoleKey.UpArrow) y--; // Move enemies and environment xEnemy += incrXEnemy; if ((xEnemy == 0) || (xEnemy == 79)) incrXEnemy = -incrXEnemy; // Collisions, lose energy or lives, etc if ((x == xDot1) && (y == yDot1) && visibleDot1) { score += 10; visibleDot1 = false; } if ((x == xDot2) && (y == yDot2) && visibleDot2) { score += 10; visibleDot2 = false; } if ((x == xDot3) && (y == yDot3) && visibleDot3) { score += 10; visibleDot3 = false; } // Pause till next fotogram // TO DO } } }
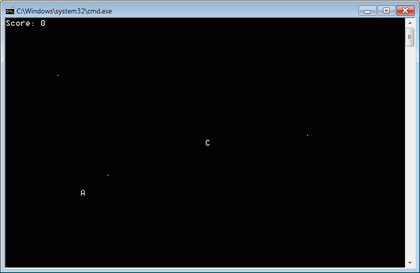
But this "is not very realistic" yet: the enemy moves, but only when our character does. We'll improve it in the next section.
Suggested exercise: Add another moving enemy.