1. Writing a symbol centered on screen
In the first parts of this tutorial, we will develop the skeleton of a basic platform game (aiming to a Pac-man-like game), but we will do it in "text mode" (using letters instead of images), so that we can start with very simple programming structures, and improve it in each step, but not worrying yet about more complex details such as image loading, animations or playing sounds.
We can use "Write" if we want to write something and not to advance to the next line on screen, or "WriteLine" if we want to advance. Therefore, if we want to write a "C" symbol resembling our "Pac-man" in the middle of the screen, we might leave a few empty lines and then write spaces followed by the desired symbol:
/* First mini-console-game skeleton Version A: "draw" the player centered, using spaces */ using System; public class Game01a { public static void Main() { Console.WriteLine(); Console.WriteLine(); Console.WriteLine(); Console.WriteLine(); Console.WriteLine(); Console.WriteLine(); Console.WriteLine(); Console.WriteLine(); Console.WriteLine(); Console.WriteLine(); Console.WriteLine(); Console.Write(" "); Console.Write("C"); } }
Which would look like this:
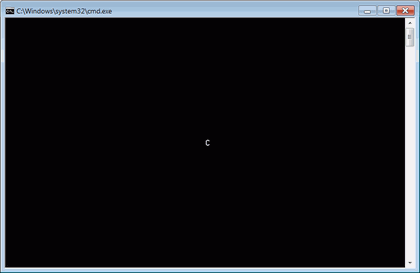
If we have the "dot net platform" version 2 or above (which is very likely to happen), we can use "Clear" to clear the screen and then "SetCursorPosition" to move the cursor to a certain position:
/* First mini-console-game skeleton Version B: "draw" the player centered, using SetCursorPosition */ using System; public class Game01b { public static void Main() { Console.Clear(); Console.SetCursorPosition(40, 12); Console.Write("C"); } }
Suggested exercise: Write the text "Score" on the top right of the screen, too.